Umbraco Elite Developers
Experience the symphony of superior Umbraco Web Development with our experts. Every line of code is composed with care, every feature orchestrated to perfection
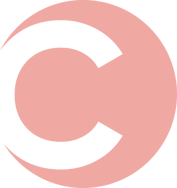
Discover our trending articles
👀Check Out Our Popular Umbraco Package
Unlock the Power of Umbraco
Turbocharge Your Media Management with Dedicated Media Folder package – The Ultimate Editor's Tool💚
