Table of contents
Introduction
Validating WordPress user passwords in .NET Core can be a complex task.
This guide provides a step-by-step approach, complete with C# code examples and unit tests using xUnit, to make this process easier and more understandable.
When to Use .NET Core and JWT Authentication for WordPress Password Validation
For Custom Authentication Systems
This approach is particularly beneficial when integrating .NET Core applications with WordPress websites that require custom authentication systems.
It allows for seamless user verification across platforms.
During WordPress API Integration
If you're developing a .NET Core application that needs to interact with WordPress's REST API, this method ensures you can authenticate WordPress users reliably within your application.
In Multi-Platform Environments
For projects where WordPress is part of a larger ecosystem involving .NET Core applications, this approach is crucial for maintaining consistent and secure user authentication across different platforms.
When Implementing Single Sign-On (SSO)
Understanding this password validation process is essential to create a single sign-on experience for users across WordPress and .NET Core applications.
For Developers Transitioning from PHP to .NET Core
This guide is handy for developers familiar with WordPress (PHP-based) and transitioning to or integrating with .NET Core technologies such as Umbraco CMS.
In Custom CMS Development
This approach allows for seamless user management and authentication when developing a custom content management system (CMS) in .NET Core that must work with a WordPress site.
Create New WordPress User Credentials
Before diving into the password validation process in .NET Core, setting up a user in WordPress to work with is essential.
We'll create a new user with the following credentials:
- Username: johndoe
- Email: johndoe@example.com
- Password: tJ)^A9t#2UrQhQVb2GAVd*9%
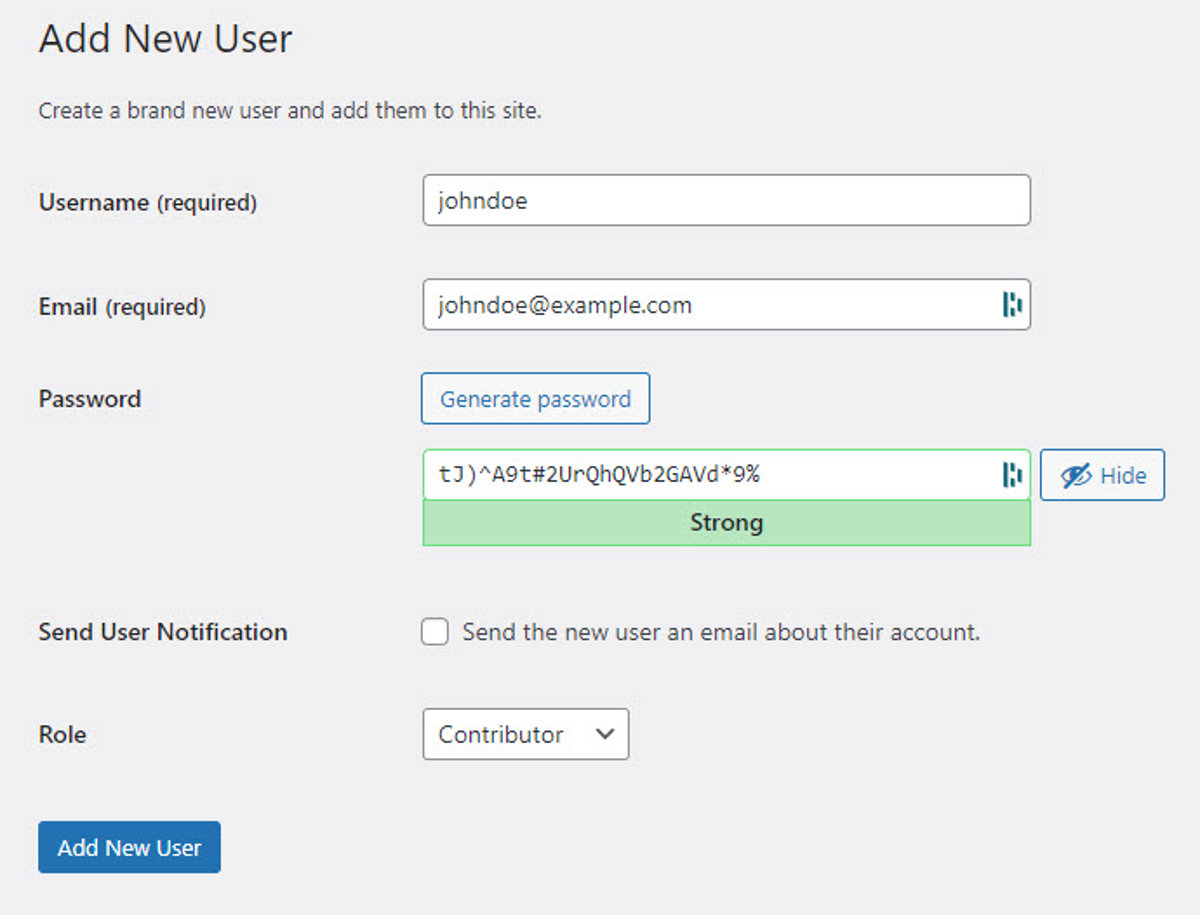
Creating a new WordPress User for integration
With 'johndoe' now set up in WordPress, we can validate this user's password through a .NET Core application.
The subsequent sections of the article will guide you through this process, ensuring a clear understanding of how to authenticate WordPress users within a .NET Core environment.
Install JWT Authentication for the WP REST API Plugin in WordPress
First and foremost, you must install and activate the JWT Authentication for WP-API plugin in your WordPress site.
This plugin is essential for enabling the REST API endpoint that facilitates authentication.
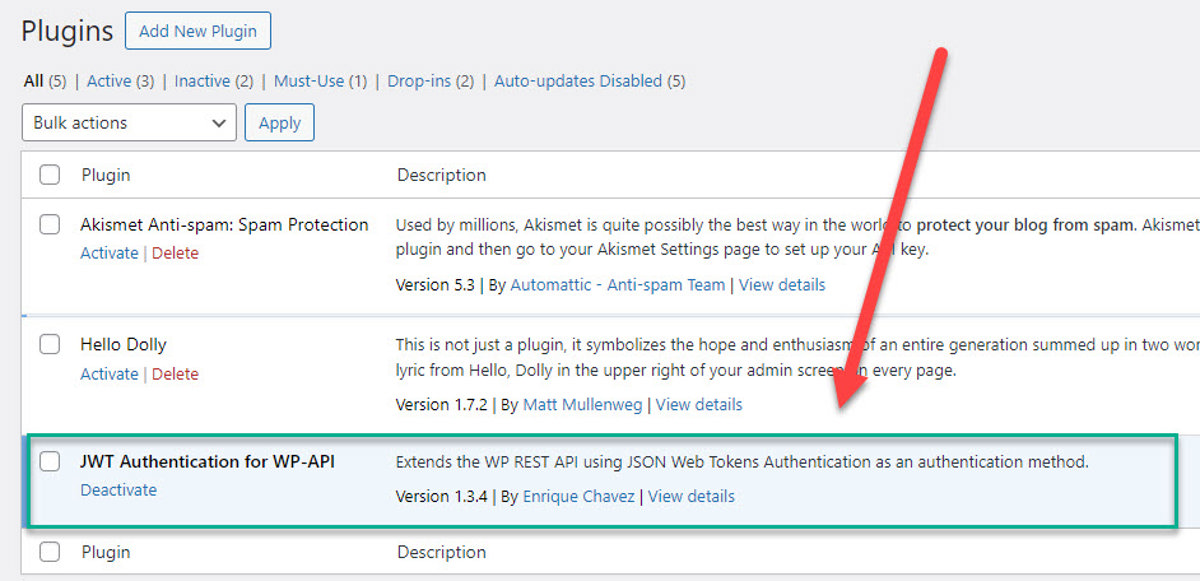
Installing JWT Authentication for the WP REST API Plugin (WordPress Admin view)
Configure wp-config.php for JWT Authentication
To ensure the JWT Authentication for the WP-API plugin works correctly with your .NET Core application, it's essential to make specific configurations in the wp-config.php file of your WordPress site.
This involves adding two key definitions:
JWT Authentication Secret Key
- Syntax: define('JWT_AUTH_SECRET_KEY', 'your-secret-key');
- Purpose: This line defines a secret key used by the JWT Authentication plugin to sign the tokens. It's a critical security feature, as it ensures that the tokens are unique and encrypted.
- Example: define('JWT_AUTH_SECRET_KEY', 'ttyox9-1H_H5v8a7X7JJxxTBEQVhcwDauuzyGANfIpKwu6duC_qKKHtKWyuMly8yKzZOhzGq0V4r61ZH4ClPiA');
- Note: The secret key should be a long, complex, and unique string to ensure maximum security.
JWT Authentication CORS Enable
- Syntax: define('JWT_AUTH_CORS_ENABLE', true);
- Purpose: This line enables Cross-Origin Resource Sharing (CORS) for the JWT Authentication API. CORS is a security feature that allows or restricts resources on a web page to be requested from another domain outside the domain from which the first resource was served.
- Importance: Setting this to true is crucial, especially if your .NET Core application is hosted on a different domain than your WordPress site. It allows the API to accept requests from your application without being blocked by the browser's Same-Origin Policy.
Steps to Edit wp-config.php:
- Access File: Locate the wp-config.php file in the root directory of your WordPress installation.
- Edit File: Open the file in a text editor.
- Add Definitions: Add the below lines of code to enable JWT Auth secret key and CORS.
- Save Changes: If you edit it locally, save the file and upload it back to the server.
Ensuring Security:
- Protect Your Secret Key: Keep your JWT secret key confidential and secure. If it's compromised, it could lead to unauthorized access.
- Regular Updates: Regularly update your secret key to maintain security.
- Backup: Always keep a backup of your original wp-config.php file before making any changes.
By editing the wp-config.php file with these configurations, you ensure that the JWT Authentication plugin operates correctly and securely, facilitating the integration with your .NET Core application.
define('JWT_AUTH_SECRET_KEY', 'ttyox9-1H_H5v8a7X7JJxxTBEQVhcwDauuzyGANfIpKwu6duC_qKKHtKWyuMly8yKzZOhzGq0V4r61ZH4ClPiA');
define('JWT_AUTH_CORS_ENABLE', true);

Configuring wp-config.php for JWT Authentication with two additional lines
Implement WordPress Authentication Class in C#
The WordPressAuthentication class plays a pivotal role.
This class contains the ValidateUserAsync method, responsible for sending the login request to the WordPress REST API and handling the response.
Code Explanation
- HttpClient Configuration: The HttpClient is configured with the base URL of your WordPress site.
- Login Request Preparation: A FormUrlEncodedContent object is created containing the username and password.
- Sending Login Request: The login request is posted to the WordPress REST API.
- Response Handling: The response is read and deserialized. If successful, the method returns true; otherwise, it returns false.
public class WordPressAuthentication
{
public static async Task<bool> ValidateUserAsync(
string username,
string password,
string wordpressSiteUrl)
{
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri(wordpressSiteUrl);
// Prepare the login request
var content = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("username", username),
new KeyValuePair<string, string>("password", password)
});
// Send the login request
HttpResponseMessage response = await client.PostAsync("/wp-json/jwt-auth/v1/token", content);
var responseContent = await response.Content.ReadAsStringAsync();
//Deserialize token
var tokenResponse = JsonSerializer.Deserialize<TokenResponse>(responseContent);
if (response.IsSuccessStatusCode)
{
// User authentication succeeded
return true;
}
else
{
// User authentication failed
return false;
}
}
}
public class TokenResponse
{
[JsonPropertyName("token")]
public string Token { get; set; }
}
}
Writing Unit Tests with xUnit
Unit tests are crucial for ensuring that your code works as expected.
The article provides an example of a test method, WhenRightCredentialsProvidedReturnTrue, which checks whether the authentication method returns true for valid credentials.
public class WordPressAuthenticationTests
{
private readonly ITestOutputHelper _testOutputHelper;
public WordPressAuthenticationTests(ITestOutputHelper testOutputHelper)
{
_testOutputHelper = testOutputHelper;
}
[Fact]
public void WhenRightCredentialsProvidedReturnTrue()
{
string username = "johndoe@example.com";
string password = "tJ)^A9t#2UrQhQVb2GAVd*9%";
string wordpressSiteUrl = "https://wordpress-734044-4080664.cloudwaysapps.com";
var wpUserPasswordIsValid = WordPressAuthentication
.ValidateUserAsync(username, password, wordpressSiteUrl)
.Result;
_testOutputHelper.WriteLine($"{username} password {password} is valid: {wpUserPasswordIsValid}");
wpUserPasswordIsValid.Should().BeTrue();
}
}
Let's break down this code to understand a unit test class called WordPressAuthenticationTests, designed to test the functionality of a WordPress authentication system in a .NET environment.
Class Declaration
public class WordPressAuthenticationTests
This line declares a new class named WordPressAuthenticationTests.
This class contains unit tests for testing WordPress authentication.
Private Field
private readonly ITestOutputHelper _testOutputHelper
This is a private field within the class.
It's used to output test results.
The readonly keyword indicates that this field can only be assigned in the constructor or at declaration.
Constructor
public WordPressAuthenticationTests(ITestOutputHelper testOutputHelper)
{
_testOutputHelper = testOutputHelper;
}
This is the constructor of the class.
It takes an ITestOutputHelper object as a parameter.
This object is used to output the test results, and it's assigned to the private field _testOutputHelper.
Test Method
[Fact] public void WhenRightCredentialsProvidedReturnTrue() { ... }:
This test method is indicated by the [Fact] attribute, which tells the testing framework that this is a unit test.
The method's name, WhenRightCredentialsProvidedReturnTrue, describes what the test is meant to verify.
In this case, the method returns true when the correct credentials are provided.
Defining Test Credentials
Inside the test method, it defines username, password, and wordpressSiteUrl with specific values.
These are the credentials and the URL of the WordPress site to be tested.
Calling the Authentication Method
The line:
var wpUserPasswordIsValid = WordPressAuthentication.ValidateUserAsync(username, password, wordpressSiteUrl).Result
calls a method ValidateUserAsync from the WordPressAuthentication class.
This method is asynchronous and checks if the given username and password are valid for the specified WordPress site.
The result (true or false) is stored in wpUserPasswordIsValid.
Outputting the Result
_testOutputHelper.WriteLine($"{username} password {password} is valid: {wpUserPasswordIsValid}");
This line outputs the test result, indicating whether the provided username and password are valid.
Assertion
wpUserPasswordIsValid.Should().BeTrue();
This assertion checks if wpUserPasswordIsValid is true.
If it's not, the test will fail.
This assertion is crucial as it confirms that the authentication method works correctly when provided with valid credentials.
In summary, this code is a unit test for verifying that the WordPress authentication method correctly validates a user when provided with the right username and password.
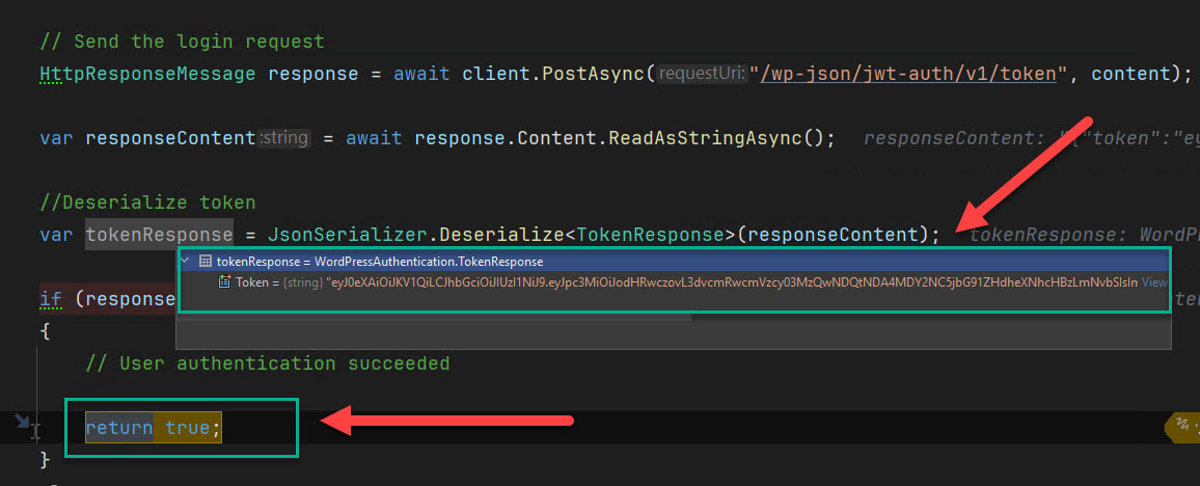
WordPress User credentials are valid (token response in runtime)

WordPress User credentials are valid - test output
This below note is crucial for developers to ensure that the integration process is smooth and that security is not compromised during the development and testing phases.
Important Note: Handling CAPTCHA in WordPress Authentication
When implementing the .NET Core method for WordPress password validation, it's crucial to be aware of the potential impact of CAPTCHA systems.
CAPTCHA, designed to distinguish human users from bots, can interfere with automated authentication processes like the one we've discussed.
Disabling CAPTCHA for Integration:
- Check for CAPTCHA: Before beginning the integration, verify if your WordPress site uses a CAPTCHA system on the login page.
- Temporarily Disable CAPTCHA: For the integration to work seamlessly, temporarily disable CAPTCHA during the development and testing phases.
- Consider Alternatives: If disabling CAPTCHA is not feasible due to security concerns, consider alternative methods such as integrating CAPTCHA handling within your .NET Core application or using API keys for authentication.
Balancing Security and Functionality:
- Re-enable After Testing: Once you have tested and confirmed that the password validation works correctly, remember to re-enable the CAPTCHA to maintain the security of your WordPress site.
- Regularly Update Security Measures: Keep your WordPress site secure by regularly updating your CAPTCHA and other security measures, ensuring they are compatible with your .NET Core integration.
Conclusion: Mastering WordPress Password Validation in .NET Core
In conclusion, understanding how to validate WordPress user passwords in a .NET Core environment is invaluable for any IT professional or developer.
This guide has walked you through the step-by-step process, from setting up a WordPress user to implementing and testing password validation using C# and xUnit.
We've covered essential aspects such as activating the necessary WordPress plugin, configuring your WordPress, and understanding the intricacies of the code involved.
Explore our blog for more insights, and feel free to reach out for any queries or discussions related to Web Development.