As far as I remember, since the ModelBuilder was introduced in Umbraco, we have been able to get an alias of any property in the easiest way as below:
ContentPage.GetModelPropertyType(x => x.Title).Alias
The funny thing is that the same solution is still presented in the current Umbraco documentation:
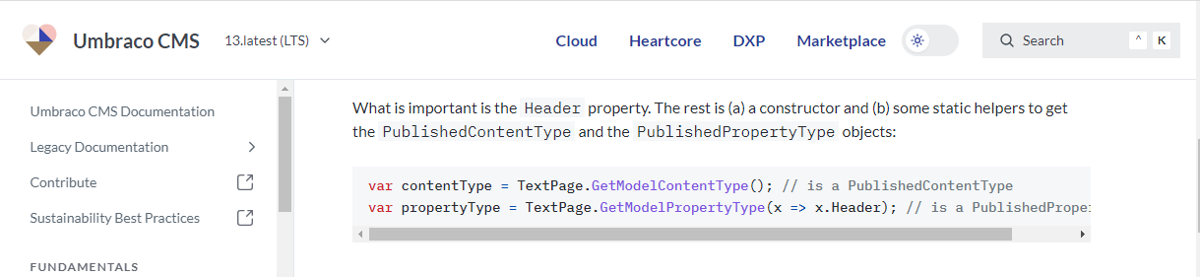
Source: https://docs.umbraco.com/umbraco-cms/reference/templating/modelsbuilder/understand-and-extend
Unfortunately, since Umbraco 10, we need the IPublishedSnapshotAccessor instance, as we can see on the same documentation page:
public static IPublishedPropertyType GetModelPropertyType<TValue>(IPublishedSnapshotAccessor publishedSnapshotAccessor, Expression<Func<TextPage, TValue>> selector)
=> PublishedModelUtility.GetModelPropertyType(GetModelContentType(publishedSnapshotAccessor), selector);
We had been used to the easiest solution, and we don't like the new one because:
- We have to inject additional IPublishedSnapshotAccessor wherever we want to use it.
- We can't use it in static methods like extensions.
- It expands the GetModelPropertyType method call a little bit.
Therefore, we decided to implement a new easy solution similar to the previous one included in the generated models.
Our solution is based on the ImplementPropertyTypeAttribute, which is added to any property in the generated models.
You can find it in any *.generated.cs files as below:
///<summary>
/// Title
///</summary>
[ImplementPropertyType("title")]
public virtual string Title => this.Value<string>(_publishedValueFallback, "title");
First of all, we implemented a simple helper as below:
public static class PublishedModelHelper
{
public static string GetPropertyAlias<T>(Expression<Func<T, object>> expression) where T : PublishedContentModel
{
var pInfo = expression.GetPropertyInfo().GetCustomAttribute<ImplementPropertyTypeAttribute>();
return pInfo.Alias;
}
}
Then implemented an additional required extension for the PropertyInfo class:
public static class ReflectionExtensions
{
public static PropertyInfo GetPropertyInfo<T>(this Expression<Func<T, object>> expression)
{
switch (expression?.Body)
{
case null:
throw new ArgumentNullException(nameof(expression));
case UnaryExpression unaryExp when unaryExp.Operand is MemberExpression memberExp:
return (PropertyInfo)memberExp.Member;
case MemberExpression memberExp:
return (PropertyInfo)memberExp.Member;
default:
throw new ArgumentException($"The expression doesn't indicate a valid property. [ {expression} ]");
}
}
}
We realized really quickly that we need additional method for PublishedElementModel:
public static string GetElementPropertyAlias<T>(Expression<Func<T, object>> expression) where T : PublishedElementModel
{
var pInfo = expression.GetPropertyInfo().GetCustomAttribute<ImplementPropertyTypeAttribute>();
return pInfo.Alias;
}
Now, we can use it as below:
string titlePropertyAlias = PublishedModelHelper.GetPropertyAlias<ContentPage>(p => p.Title);
string bodyPropertyAlias = PublishedModelHelper.GetElementPropertyAlias<QuotationBlock>(p => p.Body);
Final thoughts
The newly implemented helper for obtaining Umbraco model property aliases stands out for its ease of use and practicality.
It enhances development efficiency by avoiding complex and error-prone practices like using magic strings🙂.
Additionally, it aligns with best coding practices, fostering a more professional and scalable approach in Umbraco development.
🌐 Explore More: Interested in learning about Umbraco? Explore our blog.
✉️Get in Touch: If you have questions or need assistance with your Umbraco projects, please get in touch with us.